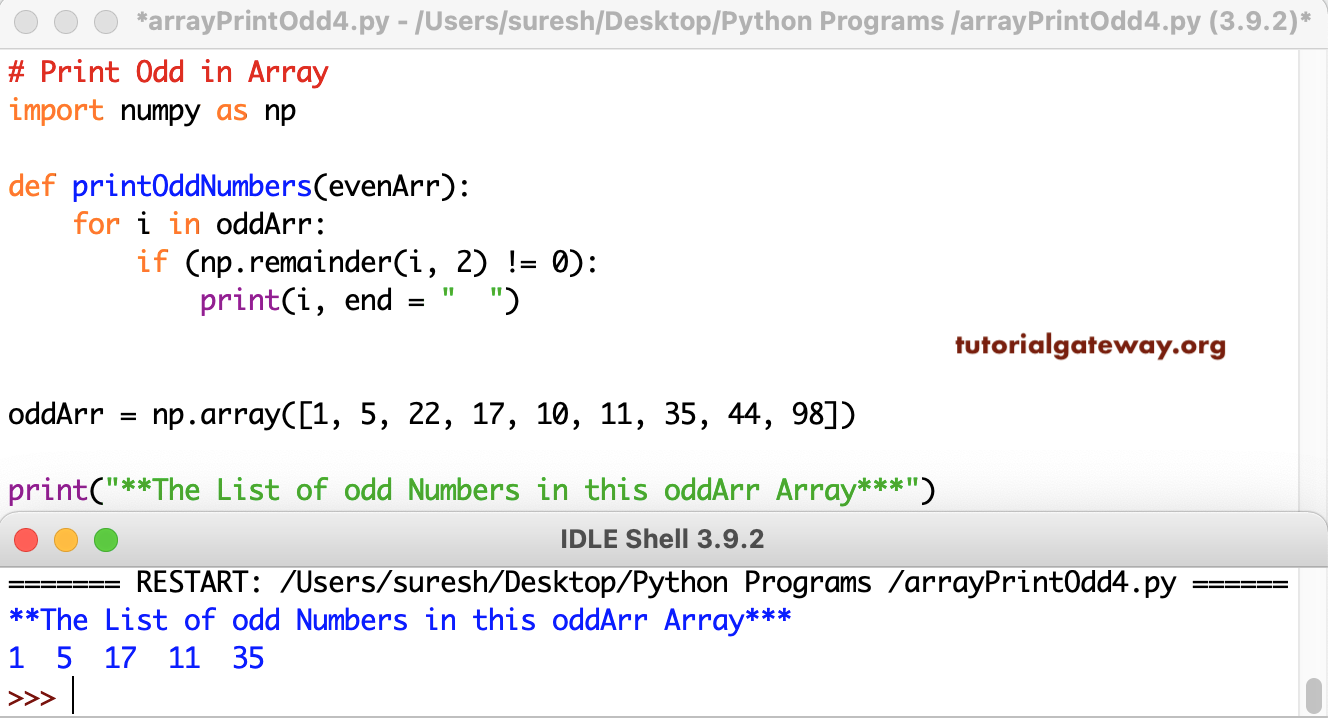
Python Program to Print Odd Numbers in an Array
Example #1: Print all odd numbers from the given list using for loop Define the start and end limit of the range. Iterate from start till the range in the list using for loop and check if num % 2 != 0. If the condition satisfies, then only print the number. Python3 start, end = 4, 19 for num in range(start, end + 1): if num % 2 != 0:
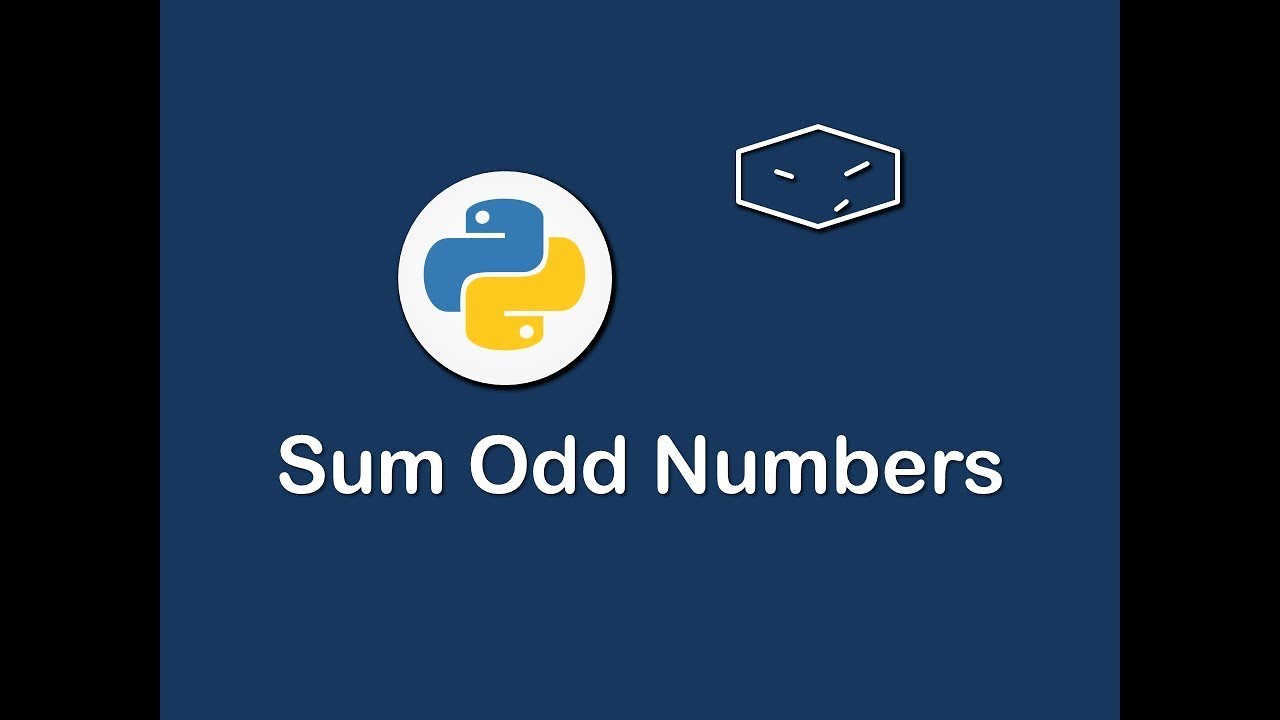
count odd numbers from a list in python ๐ YouTube
6 Answers Sorted by: 3 You were nearly there; using num % 2 is the correct method to test for odd and even numbers. return exits a function the moment it is executed. Your function returns when the first odd number is encountered. Don't use sum () if you use a loop, just add the numbers directly:

Python Program to find Sum of Even and Odd Numbers
Python Program to Check if a Number is Odd or Even Odd and Even numbers: If you divide a number by 2 and it gives a remainder of 0 then it is known as even number, otherwise an odd number. Even number examples: 2, 4, 6, 8, 10, etc. Odd number examples: 1, 3, 5, 7, 9 etc. See this example: num = int (input ("Enter a number: ")) if (num % 2) == 0:

Evens And Odds In Python CopyAssignment
Python odd numbers from list Ask Question Asked 2 years, 3 months ago Modified 2 years, 3 months ago Viewed 2k times -3 so i been giving a list with numbers, I need to grab the odd numbers from the list and sum them, the problem is that I need to only grab the first 5 odd numbers from the list on a while loop, this is what i came up with:
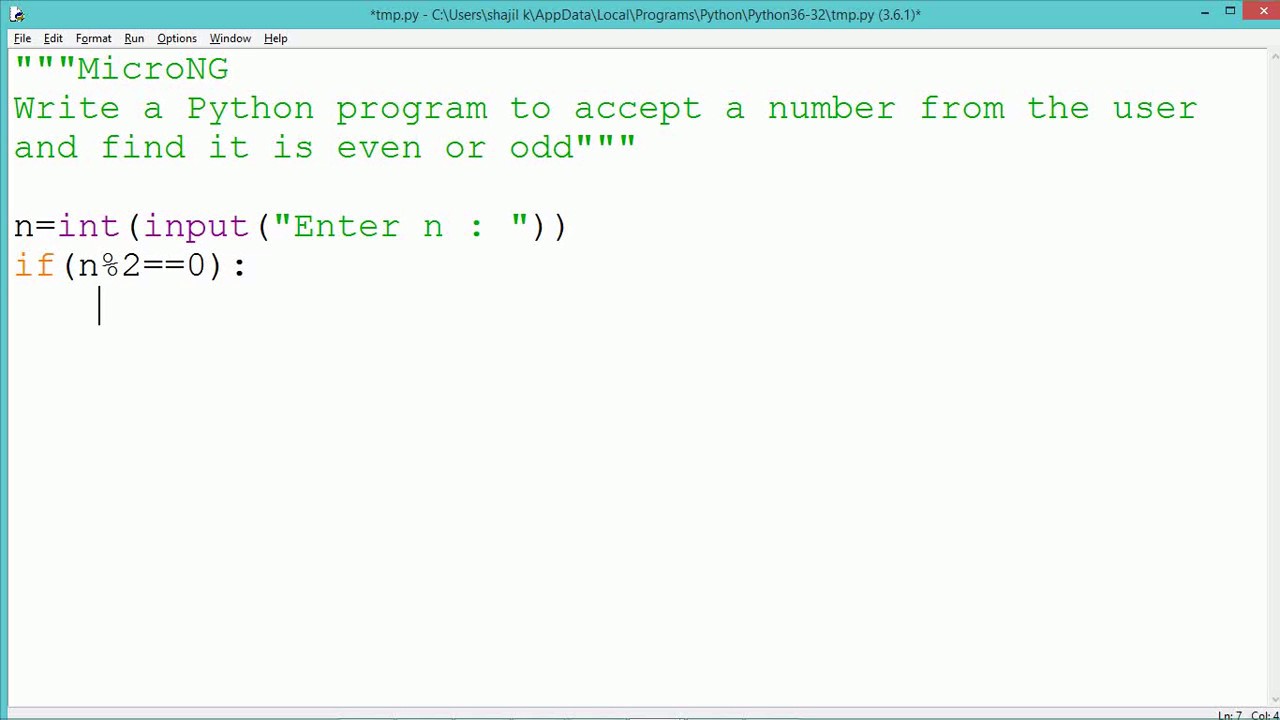
How To Check If The Number Is Even Or Odd In Python Images
Python Program to Find odd or even using function To determine whether a number is even or odd, we use the modulus operator ( % ), which returns the remainder of the division. In Python, the modulus operator is the percentage sign ( % ). When a number is divided by 2, if it leaves a remainder of 0, then it is an even number.

Python Program to Print Odd Numbers from 1 to N
if you want to print all odd numbers in one line then first you have to filter numbers and create list nums only with odd number (ie. using nums.append (number) inside for -loop) - and later print this nums (after for -loop) - furas Feb 6, 2022 at 18:24

Python Program to Find Sum of Even and Odd Numbers in an Array
Method 1: Using List Comprehension To create a list of odd numbers in Python, you can use the list comprehension [x for x in range (10) if x%2==1] that iterates over all values x between 0 and 10 (exclusive) and uses the modulo operator x%2 to check if x is divisible by 2 with remainder, i.e., x%2==1. Here's a minimal example:
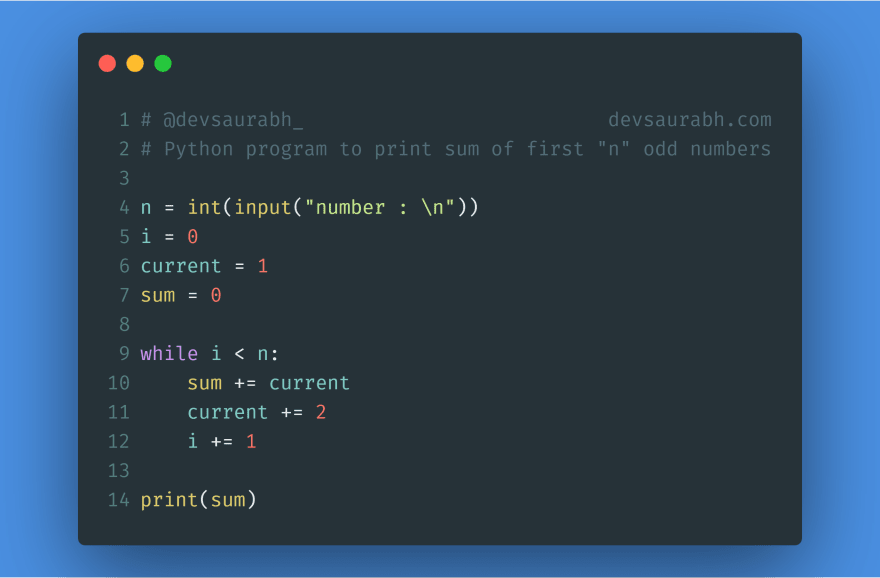
Sum of first "n" odd numbers with Python DEV Community
Output: me@amadeus:~$ python3 Templates/Aa a = 3 3 odd a = 2 2 even a = 1 1 odd a = 0 0 neutral a =. Vitalie Ghelbert is a new contributor to this site. Take care in asking for clarification, commenting, and answering. Check out our Code of Conduct. use divmod to compute remainder and division result at the same time.
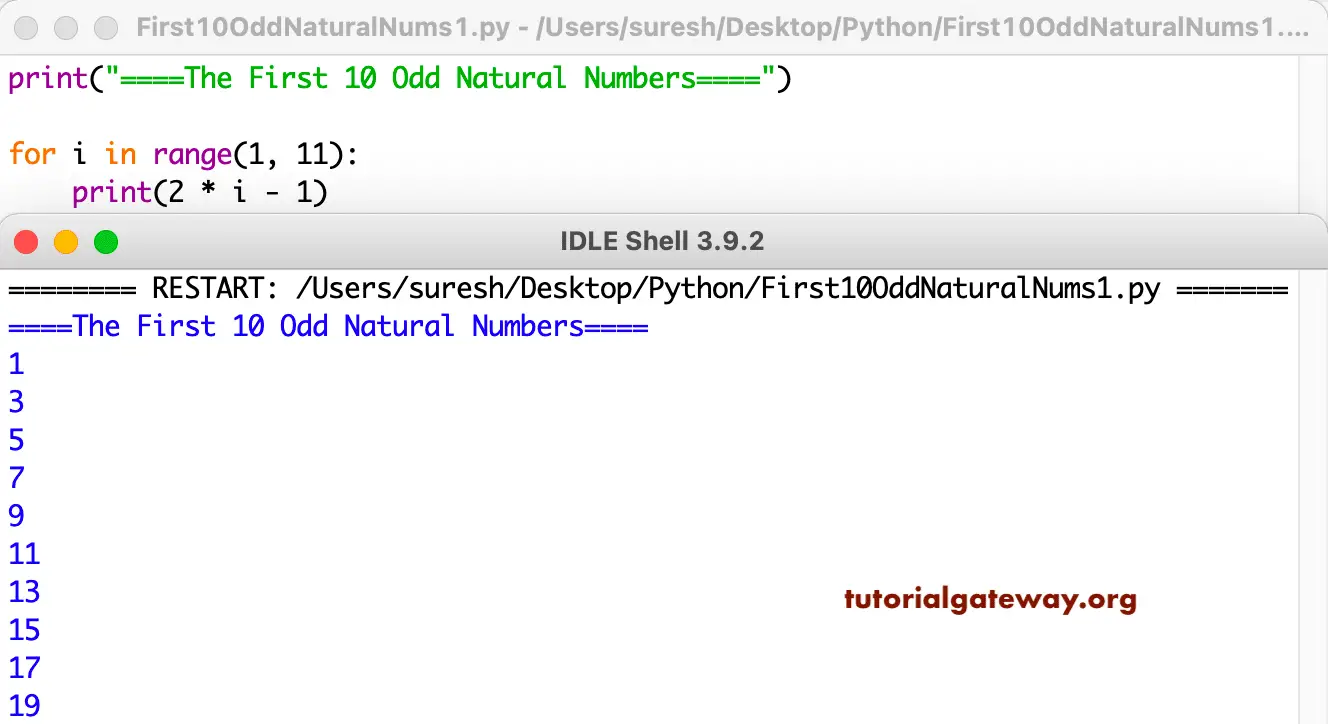
Python Program to Print First 10 Odd Natural Numbers
Explanation : This Python program uses a function to determine whether a user-inputted number is odd or even. The check_odd_even function checks for evenness using the modulo operator and returns a string indicating "even" or "odd." The user inputs a number, and the program calls the function to check its parity.

Python program to check if a number is odd or even
Given a list of numbers, write a Python program to print all odd numbers in the given list. Example: Input: list1 = [2, 7, 5, 64, 14] Output: [7, 5] Input: list2 = [12, 14, 95, 3, 73] Output: [95, 3, 73] Using for loop : Iterate each element in the list using for loop and check if num % 2 != 0.
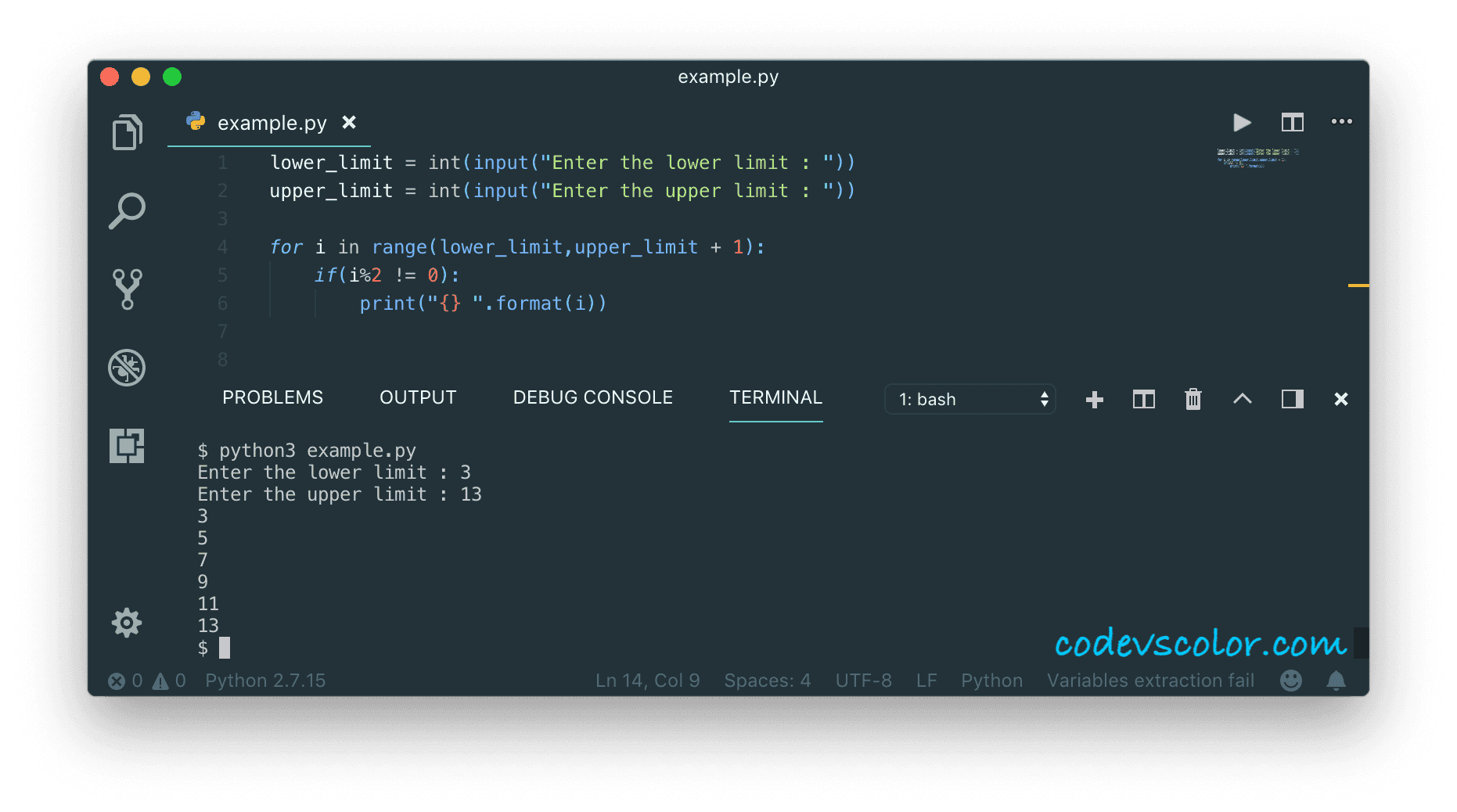
Python program to print the odd numbers in a given range CodeVsColor
Program Output: Enter a number: 6 6 is Even. If a number is evenly divisible by 2 without any remainder, then it is an even number; Otherwise, it is an odd number. The modulo operator % is used to check it in such a way as num%2 == 0. In the calculation part of the program, the given number is evenly divisible by 2 without remainder, so it is.

Python Program To Find Sum Of N Numbers With Examples Python Guides
Python Numbers. There are three numeric types in Python: int; float; complex; Variables of numeric types are created when you assign a value to them: Example. x = 1 # int y = 2.8 # float z = 1j # complex. To verify the type of any object in Python, use the type() function: Example.
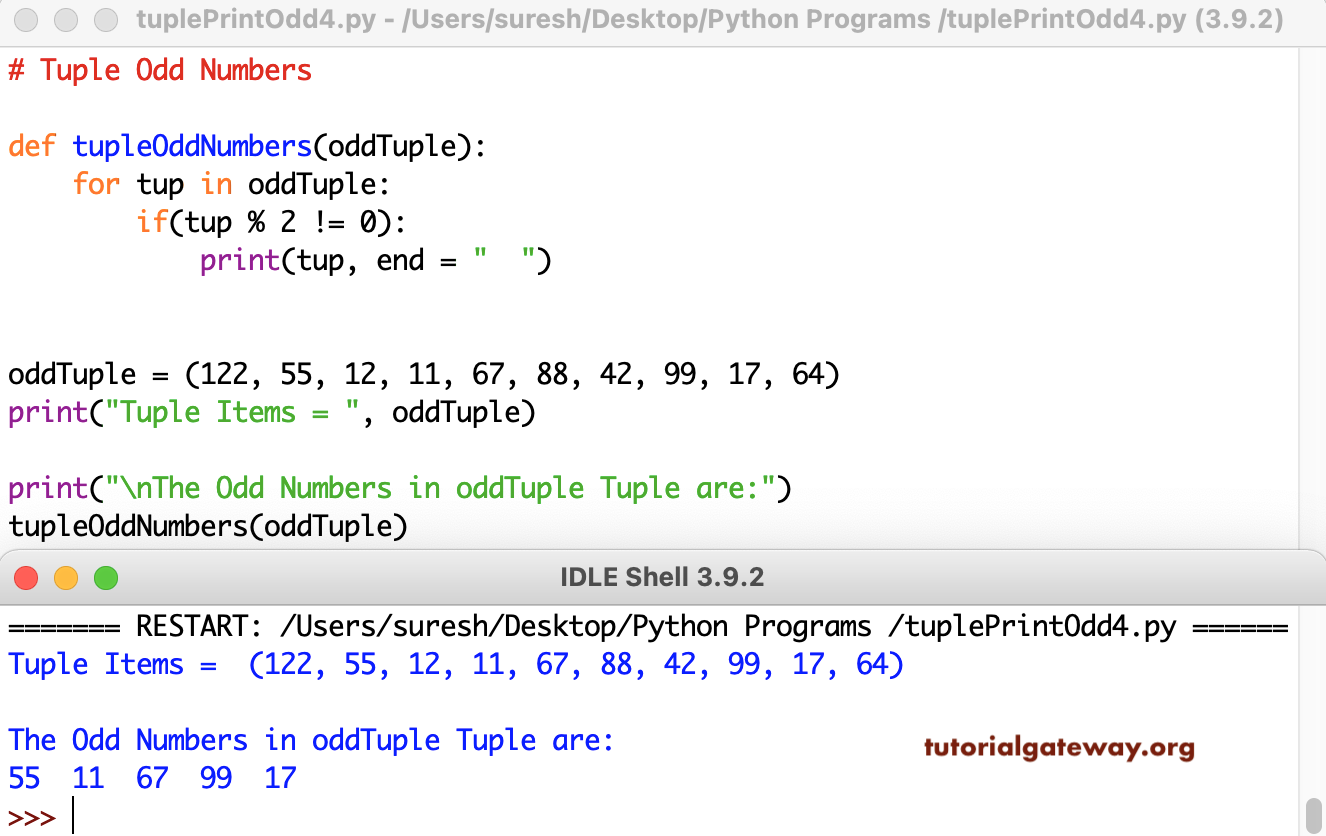
Python Program to Print Odd Numbers in Tuple
Python Operators Python if.else Statement A number is even if it is perfectly divisible by 2. When the number is divided by 2, we use the remainder operator % to compute the remainder. If the remainder is not zero, the number is odd. Source Code # Python program to check if the input number is odd or even.
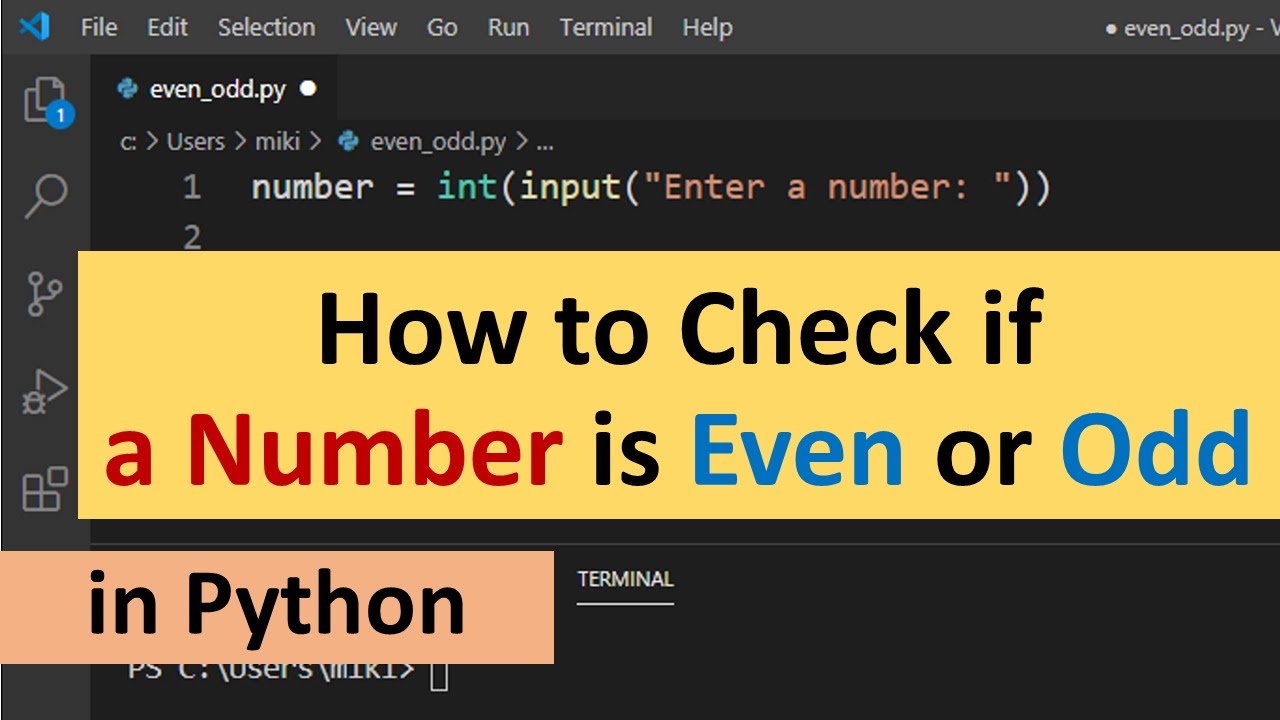
How to Check if a Number is Even or Odd in Python YouTube
While checking Python even or odd numbers, the modulus operator is used. The modulus operator returns the remainder obtained after a division is performed. If the value returned after the application of the modulus operator is zero, then the program will print that the input number is even. Else, it will print the number is odd.
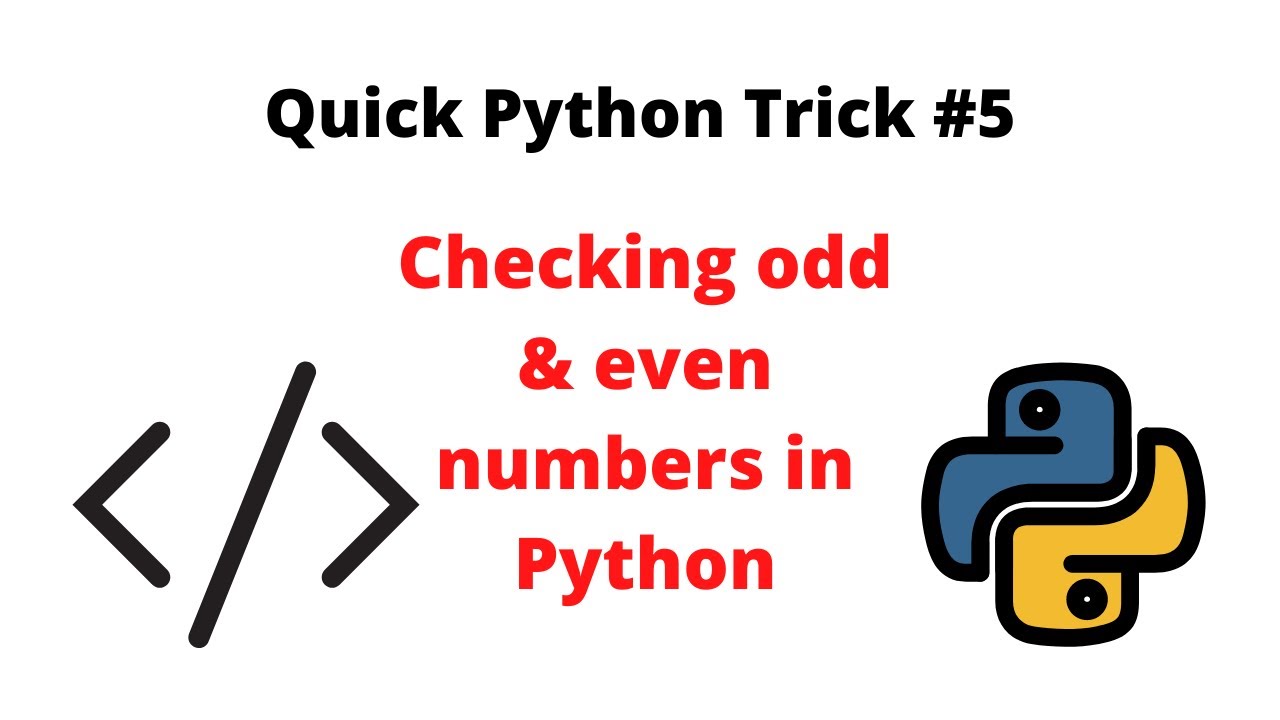
How to determine odd vs even number in Python Python tutorial Programming tips & tricks
The odd_numbers function returns a list of odd numbers between 1 and n, inclusively. Fill in the blanks in the function, using list comprehension. Hint: remember that list and range counters start at 0 and end at the limit minus 1.
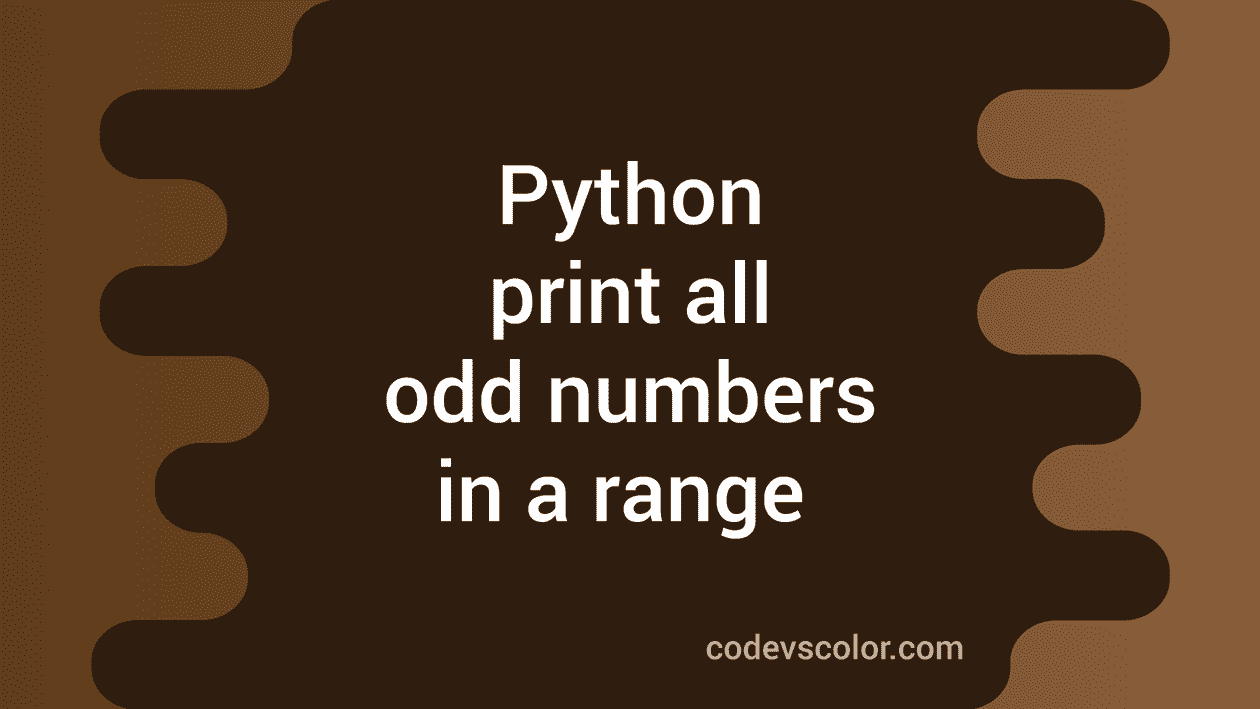
Python program to print the odd numbers in a given range CodeVsColor
Sum of Odd Numbers is calculated by adding together integers that are not divisible by 2, resulting in a total that is either an odd number or even number. Sum of Odd Numbers is often represented by the formula expressed as n 2 where n is a natural number. This formula can be used to calculate the sum of the first n odd numbers without adding them individually.